Posted At: Jul 18, 2024 - 173 Views
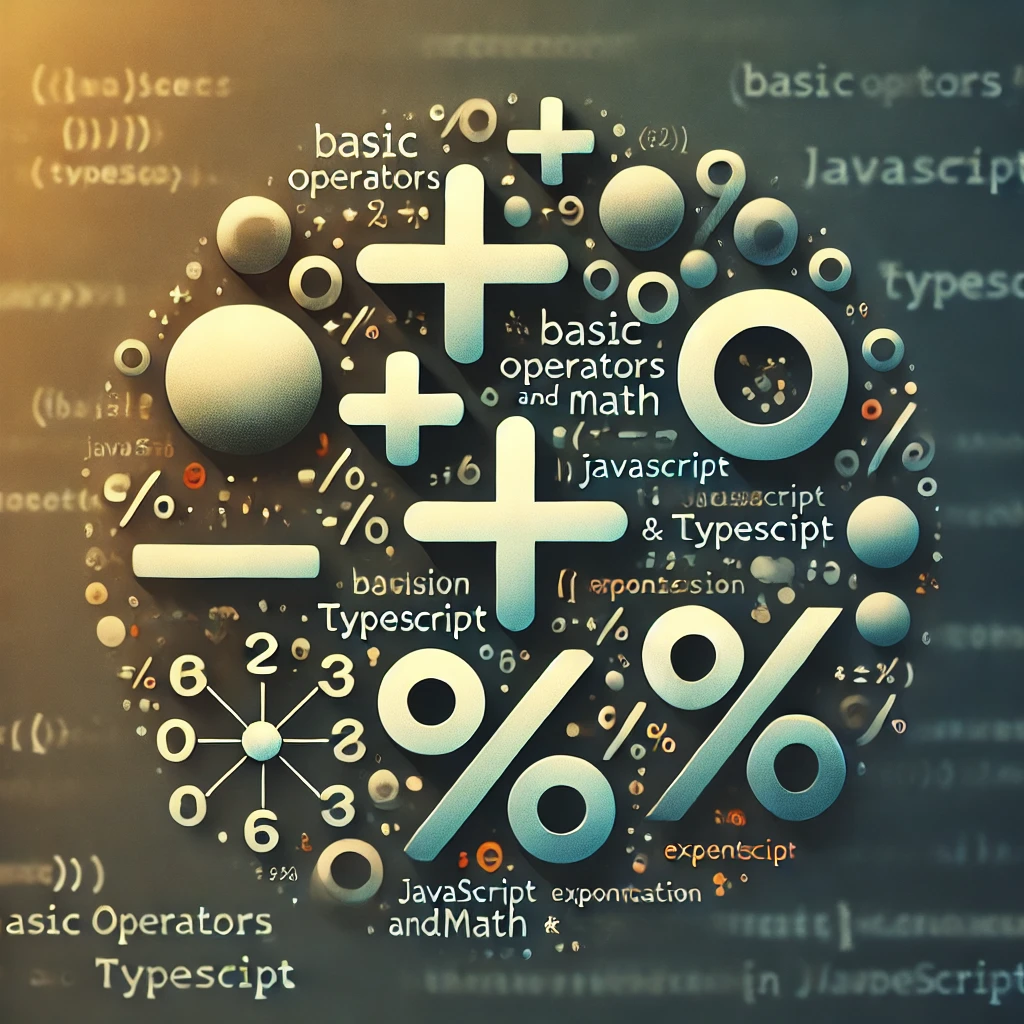
Basic Operators and Mathematics in JavaScript and TypeScript
In programming, operators such as addition (+
), multiplication (*
), subtraction (-
), and others are fundamental. This chapter covers basic operators, their applications, and JavaScript-specific nuances not typically covered in basic arithmetic.
Terms: "Unary", "Binary", "Operand"
- Operand: The entities on which operators act. For instance, in
5 * 2
,5
and2
are operands. - Unary Operator: An operator with a single operand, e.g., the unary negation (
-
) which reverses the sign of a number.
// JavaScript
let x = 5;
x = -x;
console.log(x); // -5
// TypeScript
let x: number = 5;
x = -x;
console.log(x); // -5
- Binary Operator: An operator is binary if it has two operands. The same minus exists in binary form as well:
// JavaScript
let a = 10, b = 7;
console.log(a - b); // 3
// TypeScript
let a: number = 10, b: number = 7;
console.log(a - b); // 3
Math Operations
The following math operations are supported:
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Remainder (
%
) - Exponentiation (
**
)
Remainder %
The remainder operator %
, despite its appearance, is not related to percents. The result of a % b
is the remainder of the integer division of a
by b
.
Examples:
// JavaScript
console.log(11 % 3); // 2
console.log(15 % 4); // 3
console.log(10 % 5); // 0
// TypeScript
console.log(11 % 3); // 2
console.log(15 % 4); // 3
console.log(10 % 5); // 0
Exponentiation **
The exponentiation operator a ** b
raises a
to the power of b
.
Examples:
// JavaScript
console.log(3 ** 2); // 9
console.log(2 ** 5); // 32
console.log(4 ** 0.5); // 2 (square root of 4)
// TypeScript
console.log(3 ** 2); // 9
console.log(2 ** 5); // 32
console.log(4 ** 0.5); // 2 (square root of 4)
String Concatenation with Binary +
The binary +
operator can also concatenate strings:
Examples:
// JavaScript
let greeting = "Hello, " + "World!";
console.log(greeting); // Hello, World!
// TypeScript
let greeting: string = "Hello, " + "World!";
console.log(greeting); // Hello, World!
If any operand is a string, the other one is converted to a string too:
Examples:
// JavaScript
console.log('5' + 3); // "53"
console.log(2 + '5'); // "25"
// TypeScript
console.log('5' + 3); // "53"
console.log(2 + '5'); // "25"
Here's a more complex example:
Examples:
// JavaScript
console.log(3 + 3 + '4'); // "64"
console.log('4' + 3 + 3); // "433"
// TypeScript
console.log(3 + 3 + '4'); // "64"
console.log('4' + 3 + 3); // "433"
Numeric Conversion with Unary +
The unary +
operator converts non-numbers into numbers. It does nothing to numbers.
Examples:
// JavaScript
let num1 = "7";
console.log(+num1); // 7
let bool = true;
console.log(+bool); // 1
// TypeScript
let num1: string = "7";
console.log(+num1); // 7
let bool: boolean = true;
console.log(+bool); // 1
Operator Precedence
If an expression has more than one operator, the execution order is defined by their precedence.
Examples:
// JavaScript
console.log(1 + 2 * 3); // 7 (Multiplication before addition)
console.log((1 + 2) * 3); // 9 (Parentheses change the order)
// TypeScript
console.log(1 + 2 * 3); // 7 (Multiplication before addition)
console.log((1 + 2) * 3); // 9 (Parentheses change the order)
Here's an extract from the precedence table:
Precedence | Name | Sign |
14 | Unary plus | + |
14 | Unary negation | - |
13 | Exponentiation | ** |
12 | Multiplication | * |
12 | Division | / |
11 | Addition | + |
11 | Subtraction | - |
2 | Assignment | = |
Assignment
The assignment =
operator assigns a value to a variable.
Examples:
// JavaScript
let z = 10 + 5;
console.log(z); // 15
// TypeScript
let z: number = 10 + 5;
console.log(z); // 15
Assignments can be chained:
Examples:
// JavaScript
let m, n, p;
m = n = p = 5 * 2;
console.log(m, n, p); // 10 10 10
// TypeScript
let m: number, n: number, p: number;
m = n = p = 5 * 2;
console.log(m, n, p); // 10 10 10
Modify-in-place
Short operators exist for modifying and assigning values:
Examples:
// JavaScript
let t = 4;
t += 3; // t = t + 3
console.log(t); // 7
// TypeScript
let t: number = 4;
t += 3; // t = t + 3
console.log(t); // 7
Increment/Decrement
Increment ++
increases a variable by 1, and decrement --
decreases it by 1.
Examples:
// JavaScript
let count = 0;
count++;
console.log(count); // 1
let decrement = 5;
decrement--;
console.log(decrement); // 4
// TypeScript
let count: number = 0;
count++;
console.log(count); // 1
let decrement: number = 5;
decrement--;
console.log(decrement); // 4
The prefix form returns the new value, while the postfix form returns the old value.
Examples:
// JavaScript
let val1 = 3;
let res1 = ++val1; // Prefix
console.log(res1); // 4
let val2 = 3;
let res2 = val2++; // Postfix
console.log(res2); // 3
// TypeScript
let val1: number = 3;
let res1: number = ++val1; // Prefix
console.log(res1); // 4
let val2: number = 3;
let res2: number = val2++; // Postfix
console.log(res2); // 3
Bitwise Operators
Bitwise operators work on the binary representation of numbers. They include &
, |
, ^
, ~
, <<
, >>
, >>>
.
Examples:
// JavaScript
console.log(5 & 1); // 1 (AND)
console.log(5 | 1); // 5 (OR)
console.log(5 ^ 1); // 4 (XOR)
// TypeScript
console.log(5 & 1); // 1 (AND)
console.log(5 | 1); // 5 (OR)
console.log(5 ^ 1); // 4 (XOR)
``
Comma Operator
The comma operator ,
evaluates multiple expressions and returns the result of the last one.
// JavaScript
let x = (1 + 2, 3 + 4);
console.log(x); // 7
// TypeScript
let x: number = (1 + 2, 3 + 4);
console.log(x); // 7
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com