Posted At: Jul 18, 2024 - 173 Views
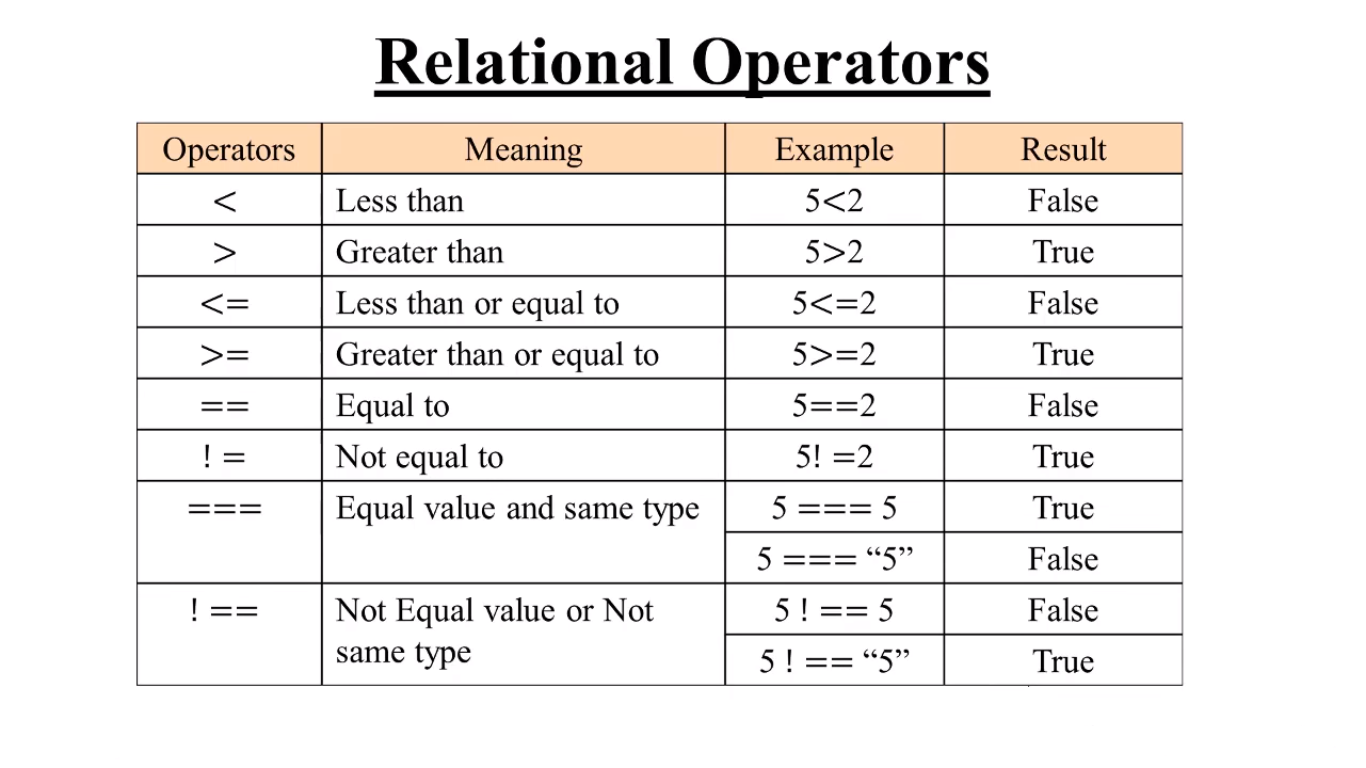
Comparisons in JavaScript and TypeScript: A Detailed Guide
In JavaScript and TypeScript, comparison operators are used to compare values. This article will explore how these comparisons work and some important peculiarities
Boolean Result
Comparison operators always return a boolean value: true
or false
.
Basic Comparison Operators
- Greater than (
>
):a > b
- Less than (
<
):a < b
- Greater than or equal to (
>=
):a >= b
- Less than or equal to (
<=
):a <= b
- Equal to (
==
):a == b
(Note: double equality==
means equality test, while a single=
means assignment) - Not equal to (
!=
):a != b
Examples:
// JavaScript
console.log(7 > 5); // true
console.log(7 == 5); // false
console.log(7 != 5); // true
// JavaScript
console.log(7 > 5); // true
console.log(7 == 5); // false
console.log(7 != 5); // true
String Comparison
Strings are compared letter-by-letter using "dictionary" or "lexicographical" order.
Algorithm for Comparing Strings:
- Compare the first character of both strings.
- If the first character from the first string is greater (or less) than the second string’s, then the first string is greater (or less) than the second. We’re done.
- Otherwise, compare the second characters the same way.
- Repeat until the end of either string.
- If both strings end at the same length, then they are equal. Otherwise, the longer string is greater.
Examples:
// JavaScript
console.log('Orange' > 'Apple'); // true: 'O' is greater than 'A'
console.log('Mango' > 'Manga'); // true: 'o' is greater than 'a'
console.log('Cat' > 'Dog'); // false: 'C' is less than 'D'
// TypeScript
console.log('Orange' > 'Apple'); // true: 'O' is greater than 'A'
console.log('Mango' > 'Manga'); // true: 'o' is greater than 'a'
console.log('Cat' > 'Dog'); // false: 'C' is less than 'D'
Comparison of Different Types
When comparing values of different types, JavaScript converts the values to numbers.
// JavaScript
console.log('15' > 10); // true: '15' is converted to 15, which is greater than 10
console.log('05' == 5); // true: '05' is converted to 5, which is equal to 5
// JavaScript
console.log('15' > 10); // true: '15' is converted to 15, which is greater than 10
console.log('05' == 5); // true: '05' is converted to 5, which is equal to 5
Boolean Values
Boolean values (true
and false
) are converted to numbers: true
becomes 1
and false
becomes 0
.
Examples:
// JavaScript
console.log(true == 1); // true: true is converted to 1, which is equal to 1
console.log(false == 0); // true: false is converted to 0, which is equal to 0
// TypeScript
console.log(true == 1); // true: true is converted to 1, which is equal to 1
console.log(false == 0); // true: false is converted to 0, which is equal to 0
A Funny Consequence:
Two values can be equal in one context but different in another.
Examples:
// JavaScript
let x = 0;
console.log(Boolean(x)); // false: 0 is converted to false
let y = "0";
console.log(Boolean(y)); // true: "0" is a non-empty string, which is true
console.log(x == y); // true: 0 and "0" are converted to 0, which are equal
// TypeScript
let x: number = 0;
console.log(Boolean(x)); // false: 0 is converted to false
let y: string = "0";
console.log(Boolean(y)); // true: "0" is a non-empty string, which is true
console.log(x == y); // true: 0 and "0" are converted to 0, which are equal
From JavaScript's standpoint, this result is normal. An equality check converts values using numeric conversion (hence "0" becomes 0), while the explicit boolean conversion uses another set of rules.
Strict Equality
A regular equality check (==
) has a problem: it cannot differentiate 0
from false
.
Examples:
// JavaScript
console.log(0 == false); // true: 0 is converted to false
console.log('' == false); // true: '' is converted to false
// JavaScript
console.log(0 == false); // true: 0 is converted to false
console.log('' == false); // true: '' is converted to false
To Differentiate 0 from False:
Use the strict equality operator ===
, which checks equality without type conversion.
Examples:
// JavaScript
console.log(0 === false); // false: 0 is not strictly equal to false
// TypeScript
console.log(0 === false); // false: 0 is not strictly equal to false
Comparison with null and undefined
Non-intuitive behavior occurs when null
or undefined
are compared to other values.
For a strict equality check (===
):
Examples:
// JavaScript
console.log(null === undefined); // false: null and undefined are different types
// TypeScript
console.log(null === undefined); // false: null and undefined are different types
For a non-strict check (==
):
Examples:
// JavaScript
console.log(null == undefined); // true: null and undefined are considered equal
// TypeScript
console.log(null == undefined); // true: null and undefined are considered equal
For mathematical and other comparisons (<
, >
, <=
, >=
):
null
is converted to 0
and undefined
to NaN
.
Examples:
// JavaScript
console.log(null > 0); // false: null is converted to 0, which is not greater than 0
console.log(null == 0); // false: null is not equal to 0
console.log(null >= 0); // true: null is converted to 0, which is equal to 0
// TypeScript
console.log(null > 0); // false: null is converted to 0, which is not greater than 0
console.log(null == 0); // false: null is not equal to 0
console.log(null >= 0); // true: null is converted to 0, which is equal to 0
An Incomparable undefined
The value undefined
shouldn’t be compared to other values:
Examples:
// JavaScript
console.log(undefined > 0); // false: undefined is converted to NaN, which is not greater than 0
console.log(undefined < 0); // false: undefined is converted to NaN, which is not less than 0
console.log(undefined == 0); // false: undefined is not equal to 0
// TypeScript
console.log(undefined > 0); // false: undefined is converted to NaN, which is not greater than 0
console.log(undefined < 0); // false: undefined is converted to NaN, which is not less than 0
console.log(undefined == 0); // false: undefined is not equal to 0
We get these results because:
- Comparisons return false because
undefined
gets converted toNaN
, which returns false for all comparisons. - The equality check returns false because
undefined
only equalsnull
,undefined
, and no other value.
Avoid Problems
- Treat any comparison with
undefined/null
except the strict equality===
with exceptional care. - Don’t use comparisons
>= > < <=
with variables that may benull/undefined
unless you’re really sure of what you’re doing. If a variable can have these values, check for them separately.
Summary
- Comparison operators return a boolean value.
- Strings are compared letter-by-letter in "dictionary" order.
- When values of different types are compared, they are converted to numbers (except for strict equality check).
null
andundefined
equal==
each other and do not equal any other value.- Be careful using comparisons like
>
or<
with variables that can benull/undefined
. Checking fornull/undefined
separately is a good practice.
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com