Posted At: Jul 18, 2024 - 191 Views
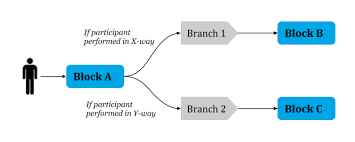
Conditional Branching: if
and ?
Sometimes, we need to perform different actions based on different conditions. To do that, we can use the if
statement and the conditional operator ?
, also known as the "question mark" operator.
The if
Statement
The if(...)
statement checks a condition in parentheses and, if the result is true, runs a block of code.
For example:
// JavaScript
let favoriteFruit = prompt('What is your favorite fruit?', '');
if (favoriteFruit === 'apple') {
alert('Apples are delicious!');
} else if (favoriteFruit === 'banana') {
alert('Bananas are great for a quick snack!');
} else {
alert(`Wow, ${favoriteFruit} is a unique choice!`);
}
// TypeScript
let favoriteFruit: string | null = prompt('What is your favorite fruit?', '');
if (favoriteFruit === 'apple') {
alert('Apples are delicious!');
} else if (favoriteFruit === 'banana') {
alert('Bananas are great for a quick snack!');
} else {
alert(`Wow, ${favoriteFruit} is a unique choice!`);
}
In this example, the condition checks the value of favoriteFruit
. Depending on the value, it displays a different message.
When we need to run more than one statement, we use curly braces:
// JavaScript
if (favoriteFruit === 'apple') {
alert('Apples are delicious!');
alert("They're also great in pies!");
}
// TypeScript
if (favoriteFruit === 'apple') {
alert('Apples are delicious!');
alert("They're also great in pies!");
}
Always using curly braces {}
helps with readability, even if there's only one statement.
Boolean Conversion
The if (...)
statement evaluates the expression in its parentheses and converts the result to a boolean.
Conversion rules:
- Falsy values:
0
,""
(empty string),null
,undefined
, andNaN
- Truthy values: All other values
Examples:
// JavaScript
if (0) {
// This code will not run
}
if (1) {
// This code will run
}
// TypeScript
if (0) {
// This code will not run
}
if (1) {
// This code will run
}
We can also pass a pre-evaluated boolean value to if
:
// JavaScript
let age = 25;
let isAdult = (age >= 18);
if (isAdult) {
alert('You are an adult!');
}
// TypeScript
let age: number = 25;
let isAdult: boolean = (age >= 18);
if (isAdult) {
alert('You are an adult!');
}
The else
Clause
The if
statement can have an optional else
block, which runs when the condition is false.
// JavaScript
let weather = prompt('Is it raining?', '');
if (weather === 'yes') {
alert('Take an umbrella!');
} else {
alert('Enjoy the sunshine!');
}
// TypeScript
let weather: string | null = prompt('Is it raining?', '');
if (weather === 'yes') {
alert('Take an umbrella!');
} else {
alert('Enjoy the sunshine!');
}
Several Conditions: else if
The else if
clause lets us check multiple conditions.
// JavaScript
let time = prompt('What time is it (0-23)?', '');
if (time < 12) {
alert('Good morning!');
} else if (time < 18) {
alert('Good afternoon!');
} else if (time < 22) {
alert('Good evening!');
} else {
alert('Good night!');
}
// TypeScript
let time: string | null = prompt('What time is it (0-23)?', '');
if (time !== null) {
let numTime: number = parseInt(time);
if (numTime < 12) {
alert('Good morning!');
} else if (numTime < 18) {
alert('Good afternoon!');
} else if (numTime < 22) {
alert('Good evening!');
} else {
alert('Good night!');
}
}
Conditional Operator ?
The conditional (ternary) operator allows us to assign a value based on a condition.
// JavaScript
let temperature = prompt('What is the temperature?', '');
let isCold = temperature < 15 ? true : false;
alert(isCold ? 'Brrr! It’s cold!' : 'Nice weather!');
// TypeScript
let temperature: string | null = prompt('What is the temperature?', '');
let isCold: boolean = temperature !== null && parseInt(temperature) < 15 ? true : false;
alert(isCold ? 'Brrr! It’s cold!' : 'Nice weather!');
Multiple ?
We can use multiple conditional operators to return a value based on several conditions.
// JavaScript
let age = prompt('How old are you?', '');
let message = (age < 5) ? 'Awww, so young!' :
(age < 13) ? 'Hey there, kiddo!' :
(age < 20) ? 'What’s up, teenager?' :
(age < 30) ? 'Hello, young adult!' :
(age < 65) ? 'Greetings, adult!' :
'Respect, senior citizen!';
alert(message);
// TypeScript
let age: string | null = prompt('How old are you?', '');
let message: string = age !== null ? (
parseInt(age) < 5 ? 'Awww, so young!' :
parseInt(age) < 13 ? 'Hey there, kiddo!' :
parseInt(age) < 20 ? 'What’s up, teenager?' :
parseInt(age) < 30 ? 'Hello, young adult!' :
parseInt(age) < 65 ? 'Greetings, adult!' :
'Respect, senior citizen!'
) : 'Invalid age';
alert(message);
Non-traditional Use of ?
The question mark ?
can replace if
, but it is generally less readable.
// JavaScript
let answer = prompt('Do you like JavaScript?', '');
answer === 'yes' ? alert('Great!') : alert('Oh no, why not?');
// JavaScript
let answer = prompt('Do you like JavaScript?', '');
answer === 'yes' ? alert('Great!') : alert('Oh no, why not?');
For better readability, use if
instead:
// JavaScript
let answer = prompt('Do you like JavaScript?', '');
if (answer === 'yes') {
alert('Great!');
} else {
alert('Oh no, why not?');
}
// JavaScript
let answer = prompt('Do you like JavaScript?', '');
if (answer === 'yes') {
alert('Great!');
} else {
alert('Oh no, why not?');
}
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com