Posted At: Aug 03, 2024 - 116 Views
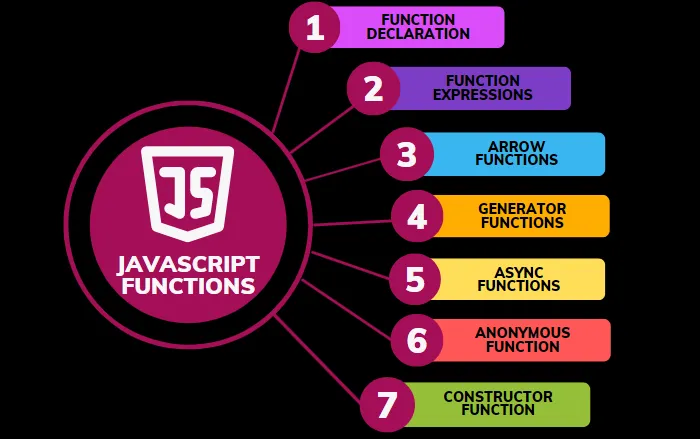
Functions in JavaScript and TypeScript
To declare a function, use the function
keyword followed by the name of the function, parameters, and the function body.
JavaScript Example:
function greet() {
console.log('Hello, World!');
}
greet(); // Output: Hello, World!
TypeScript Example:
function greet(): void {
console.log('Hello, World!');
}
greet(); // Output: Hello, World!
Parameters
Functions can take parameters to work with. These are specified within the parentheses.
JavaScript Example:
function greetUser(name) {
console.log(`Hello, ${name}!`);
}
greetUser('Alice'); // Output: Hello, Alice!
TypeScript Example:
function greetUser(name: string): void {
console.log(`Hello, ${name}!`);
}
greetUser('Alice'); // Output: Hello, Alice!
Default Parameters
Parameters can have default values which are used if no argument is passed.
JavaScript Example:
function greetUser(name = 'Guest') {
console.log(`Hello, ${name}!`);
}
greetUser(); // Output: Hello, Guest!
TypeScript Example:
function greetUser(name: string = 'Guest'): void {
console.log(`Hello, ${name}!`);
}
greetUser(); // Output: Hello, Guest!
Returning Values
Functions can return values using the return
statement.
JavaScript Example:
function add(a, b) {
return a + b;
}
console.log(add(2, 3)); // Output: 5
TypeScript Example:
function add(a: number, b: number): number {
return a + b;
}
console.log(add(2, 3)); // Output: 5
Local and Global Variables
Variables declared inside a function are local and can only be accessed within that function. Variables declared outside any function are global.
JavaScript Example:
let userName = 'Alice';
function greetUser() {
let message = `Hello, ${userName}`;
console.log(message);
}
greetUser(); // Output: Hello, Alice
TypeScript Example:
let userName: string = 'Alice';
function greetUser(): void {
let message: string = `Hello, ${userName}`;
console.log(message);
}
greetUser(); // Output: Hello, Alice
Example with Overriding Variables
JavaScript Example:
let userName = 'Alice';
function greetUser() {
let userName = 'Bob'; // Local variable
console.log(`Hello, ${userName}`); // Output: Hello, Bob
}
greetUser();
console.log(userName); // Output: Alice
TypeScript Example:
let userName: string = 'Alice';
function greetUser(): void {
let userName: string = 'Bob'; // Local variable
console.log(`Hello, ${userName}`); // Output: Hello, Bob
}
greetUser();
console.log(userName); // Output: Alice
Function Naming Conventions
- Functions are actions, so their names usually contain verbs.
- Common prefixes:
get...
: returns a value.calc...
: calculates something.create...
: creates something.check...
: checks something and returns a boolean.
Example with Naming Conventions
JavaScript Example:
function calculateSum(a, b) {
return a + b;
}
console.log(calculateSum(5, 7)); // Output: 12
TypeScript Example:
function calculateSum(a: number, b: number): number {
return a + b;
}
console.log(calculateSum(5, 7)); // Output: 12
Summary
- Functions help avoid code repetition.
- Functions can accept parameters and return values.
- Local variables are accessible only within the function they are declared.
- Global variables are accessible from any function.
- Default parameters provide fallback values.
- Naming functions appropriately makes code more readable and maintainable.
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com