Posted At: Jul 14, 2024 - 316 Views
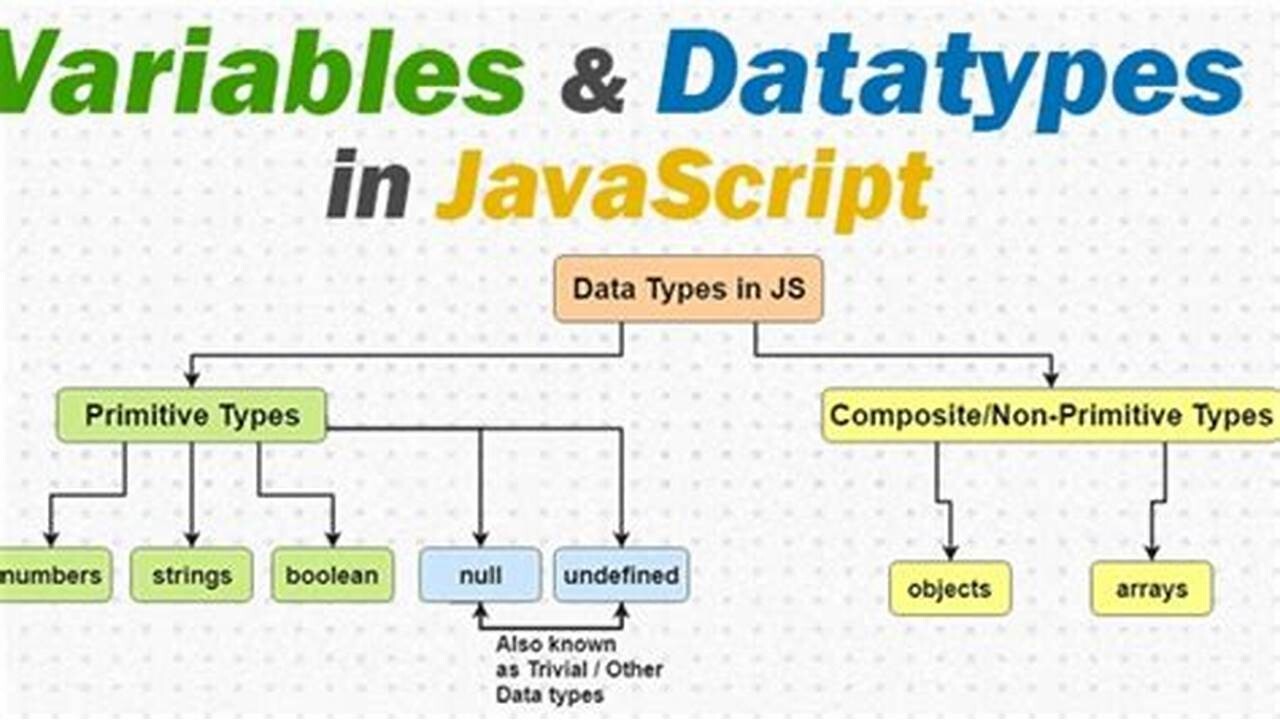
JavaScript Data Types
In JavaScript, every value has a specific type, such as a string or a number. There are eight basic data types. We'll cover them briefly here and explore each one in more detail in later sections.
Dynamic Typing
JavaScript is dynamically typed, meaning variables can hold any type of data and can change types at runtime.
let item = "Hello";
item = 12345; // No error
Types of Data
1. Number
- Represents both integers and floating-point numbers.
- Operations: multiplication
*
, division/
, addition+
, subtraction-
, etc. - Special values:
Infinity
,-Infinity
, andNaN
.
let age = 30;
let price = 12.99;
alert(1 / 0); // Infinity
alert("text" / 2); // NaN
2. BigInt
- Used for very large integers beyond the safe integer range.
- Created by appending
n
to the end of the number.
Example:
const bigNumber = 1234567890123456789012345678901234567890n;
3. String
- Must be surrounded by quotes: double
"
, single'
, or backticks`
. - Backticks allow embedding variables and expressions.
Examples:
let greeting = "Hello";
let singleQuote = 'Single quotes work too';
let name = `Aakash`;
alert(`Hello, ${name}!`); // Hello, Aakash!
4. Boolean
- Represents logical values:
true
orfalse
.
Example:
let isCompleted = true;
let isVerified = false;
let isGreater = 5 > 3;
alert(isGreater); // true
5. Null
- Represents "nothing" or "unknown value".
Example:
let user = null;
6. Undefined
- Represents an uninitialized variable.
Example:
let task;
alert(task); // undefined
7. Object
- Used to store collections of data and more complex entities.
Example:
let user = {
name: "Aakash",
age: 21
};
8. Symbol
- Used to create unique identifiers for objects.
Example:
let id = Symbol("id");
The typeof
Operator
- Returns the type of a value as a string.
- Useful for checking the type of a variable.
Examples:
typeof undefined // "undefined"
typeof 42 // "number"
typeof 1234567890123456789012345678901234567890n // "bigint"
typeof true // "boolean"
typeof "Hello" // "string"
typeof Symbol("id") // "symbol"
typeof user // "object"
typeof null // "object" (a known issue in JavaScript)
typeof alert // "function"
Summary
- Primitive Data Types:
number
,bigint
,string
,boolean
,null
,undefined
,symbol
. - Non-Primitive Data Type:
object
. - Dynamic Typing: Variables can store any type of value.
- Special Values:
Infinity
,-Infinity
,NaN
fornumber
. - Checking Types: Use
typeof
to determine the type of a variable.
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com