Posted At: Jul 08, 2024 - 158 Views
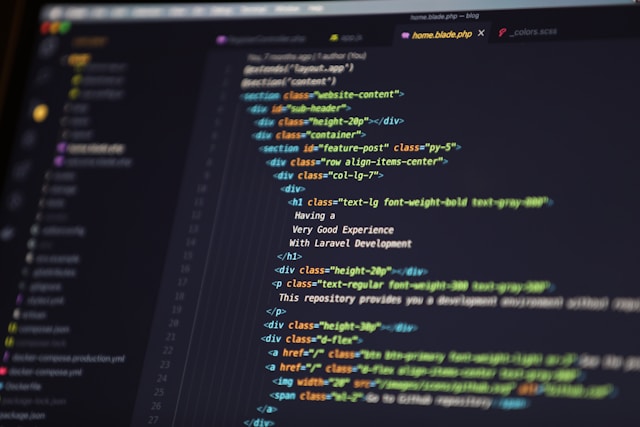
Hello, world!
This segment of the tutorial is dedicated to core JavaScript, focusing solely on the language.
Preparing the Environment
To execute our JavaScript scripts, we need a suitable environment. Since this guide is online, we’ll use the browser for our examples. We'll limit the use of browser-specific commands (like alert
) so you can apply these skills in other environments, such as Node.js. We’ll cover JavaScript in the browser in detail in the following sections.
Adding JavaScript to a Webpage
First, let’s learn how to add a JavaScript script to an HTML document. If you're using a server-side environment like Node.js, you can run your script with a command like node my.js
.
Using the <script>
Tag
You can insert JavaScript code almost anywhere within an HTML document using the <script>
tag. Here’s an example:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Example</title>
</head>
<body>
<h1>Hello, world!</h1>
<script>
console.log('Hello, world!');
</script>
</body>
</html>
In this snippet, the JavaScript code within the <script>
tag will execute when the webpage loads, displaying "Hello, world!" in the console.
Embedding JavaScript in this manner allows for the creation of interactive and dynamic web pages. This approach also facilitates easy testing and debugging directly in the browser.
Modern Markup
The <script>
tag has evolved, and some attributes that were once essential are now largely outdated. However, they can still appear in legacy code.
The type
Attribute
- Historical Context: In the older HTML4 standard, the
type
attribute was mandatory for scripts and typically set totype="text/javascript"
. - Modern Use: Today, this attribute is unnecessary. The modern HTML standard uses
type
for JavaScript modules, which we'll cover in an advanced section.
The language
Attribute
- Historical Context: This attribute was used to declare the scripting language.
- Modern Use: It has become obsolete since JavaScript is the default scripting language for browsers.
Comments in Scripts
- Historical Context: In old tutorials and guides, you might find comments embedded within
<script>
tags to hide JavaScript from browsers that didn’t recognize the tag.
<script type="text/javascript"><!--
// JavaScript code
//--></script>
- Modern Use: Such comments are no longer needed, as modern browsers understand the
<script>
tag. This practice indicates very old code.
External Scripts
For larger JavaScript codebases, it's best to separate your scripts into different files. This improves the readability and maintainability of your HTML.
Linking External Scripts
- Basic Usage: Use the
src
attribute to link to external script files.
<script src="/abc/script.js"></script>
- Absolute Path: The path starts from the root of the site.
- Relative Path: Paths like
src="script.js"
orsrc="./script.js"
reference files relative to the current directory. - Full URLs: You can link to external libraries hosted online.
<script src="https://google.com"></script>
Including Multiple Scripts
Use multiple <script>
tags to include various script files.
<script src="/sbcd/scriptone.js"></script>
<script src="/sss/scripttwo.js"></script>
Best Practices
- Separate Files for Complex Scripts: Keep complex scripts in separate files rather than embedding them directly in HTML.
- Caching Benefits: Browsers cache external files, reducing load times for returning visitors.
Script Content vs. src
Attribute
- Exclusive Usage: A
<script>
tag cannot simultaneously have ansrc
attribute and inline code.
<script src="file.js">
// This inline code will be ignored because `src` is set
</script>
- Correct Approach: Use separate
<script>
tags for the source file and the inline script.
<script src="file.js"></script>
<script>
alert('Hello!');
</script>
Understanding these practices helps in writing cleaner and more efficient JavaScript while also making it easier to recognize and handle legacy code.
Next → Code editors
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com