Posted At: Jul 13, 2024 - 356 Views
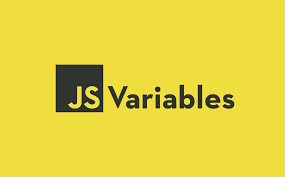
Understanding Variables in JavaScript
What are Variables?
- Definition: Variables are containers for storing data values.
- Purpose: They help manage and manipulate data within applications, such as user information in a chat app or product details in an online store.
How to Create Variables
Declaration: Use the let
keyword to declare a variable.
let message;
Initialization: Assign a value to the variable using the =
operator.
let message = 'Hello!';
Using Variables
Accessing Data: Retrieve the value stored in a variable by using its name.
let greeting = 'Hello, World!';
alert(greeting); // Displays 'Hello, World!'
Multiple Declarations
- Single Line Declaration: You can declare multiple variables in one line, but it's clearer to separate them.
let user = 'John', age = 30, message = 'Hello';
- Preferred Method: Declare each variable on a separate line for better readability.
let user = 'John';
let age = 30;
let message = 'Hello';
The var
Keyword
- Older Syntax:
var
is an older way to declare variables and has different scoping rules compared tolet
.
var message = 'Hello';
Real-Life Analogy
- Boxes with Labels: Think of variables as boxes with labels that store different items
let fruit = 'Apple';
fruit = 'Banana'; // The box labeled 'fruit' now holds 'Banana'
alert(fruit); // Displays 'Banana'
Variable Naming Rules
- Allowed Characters: Variable names can include letters, digits,
$
, and_
, but cannot start with a digit.
let userName;
let $amount = 100;
let _temp = 'temporary';
- Case Sensitivity: Variable names are case-sensitive, so
apple
andAPPLE
are different. - CamelCase Convention: Use camelCase for multi-word variable names.
let firstName = 'John';
let lastName = 'Doe';
Reserved Words
- Restricted Names: Certain words are reserved by JavaScript and cannot be used as variable names.
let let = 5; // Error: 'let' is reserved
let return = 5; // Error: 'return' is reserved
Constants
- Declaration: Use
const
to declare a variable whose value should not change
const myBirthday = '18.04.1982';
myBirthday = '01.01.2001'; // Error: Assignment to constant variable
Changing Variable Values
- Reassigning Values: Variables declared with
let
can be reassigned.
let color = 'red';
color = 'blue'; // The value of 'color' is now 'blue'
Copying Values Between Variables
- Example: Copy data from one variable to another.
let greeting = 'Hello, World!';
let message = greeting; // 'message' now holds 'Hello, World!'
alert(message); // Displays 'Hello, World!'
Variable Scope
- Function Scope: Variables declared inside a function are local to that function
function sayHello() {
let greeting = 'Hello, World!';
alert(greeting); // Displays 'Hello, World!'
}
sayHello();
alert(greeting); // Error: 'greeting' is not defined
- Block Scope: Variables declared with
let
orconst
inside a block are local to that block.
if (true) {
let message = 'Hello, World!';
alert(message); // Displays 'Hello, World!'
}
alert(message); // Error: 'message' is not defined
Declaring Twice Triggers an Error
- Single Declaration: A variable should be declared only once. Re-declaring a variable causes an error.
let message = "This";
let message = "That"; // SyntaxError: 'message' has already been declared
Functional Languages
- Immutability: Some programming languages, like Haskell, do not allow variables to change once they are declared. JavaScript allows variables to change values.
Variable Naming Rules
- Valid Characters: Variable names can contain letters, digits, underscores (_), and dollar signs ($), but must not start with a digit.
- Case Sensitivity: Variable names are case-sensitive. For example,
apple
andApple
are different variables. - Camel Case: Use camelCase for multi-word variable names. For example:
myVariableName
.
Examples of Valid Names:
let userName;
let test123;
let $ = 1;
let _ = 2;
Examples of Invalid Names:
let 1a; // Cannot start with a digit
let my-name; // Hyphens are not allowed
Reserved Words
- Restricted Names: Certain words are reserved by the JavaScript language and cannot be used as variable names. Examples include
let
,class
,return
, andfunction
.
Example:
let let = 5; // Error
let return = 5; // Error
Assigning Without Declaration
- Bad Practice: Normally, you need to declare a variable before using it. However, it is technically possible to create a variable by simply assigning a value to it without using
let
,var
, orconst
. This is generally considered bad practice and should be avoided.
Example Without use strict
:
num = 5; // Variable "num" is created if it didn't exist
alert(num); // 5
Example With use strict
:
"use strict";
num = 5; // Error: num is not defined
Constants
- Use
const
: Declare constants withconst
to prevent reassignment.
const myBirthday = '18.04.1982';
myBirthday = '01.01.2001'; // Error: Assignment to constant variable
Uppercase Constants
- Naming Convention: Use uppercase letters and underscores for constant values that are known before execution.
const COLOR_RED = "#F00";
const COLOR_GREEN = "#0F0";
const COLOR_BLUE = "#00F";
const COLOR_ORANGE = "#FF7F00";
Naming Things Right
- Descriptive Names: Use clear, descriptive names for variables to make the code more readable.
let userName = 'John';
let userAge = 25;
let userLocation = 'New York';
Reuse or Create?
- Avoid Reusing: Do not reuse variables for different purposes. Declare new variables to keep the code clean and understandable.
let userName = 'John';
let userAge = 25;
let userLocation = 'New York';
Summary
- Keywords: Use
let
,var
, orconst
to declare variables. - Naming: Use meaningful and descriptive names.
- Constants: Use
const
for values that do not change. - Scope: Understand the scope of your variables (function or block).
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com