Posted At: Jul 23, 2024 - 381 Views
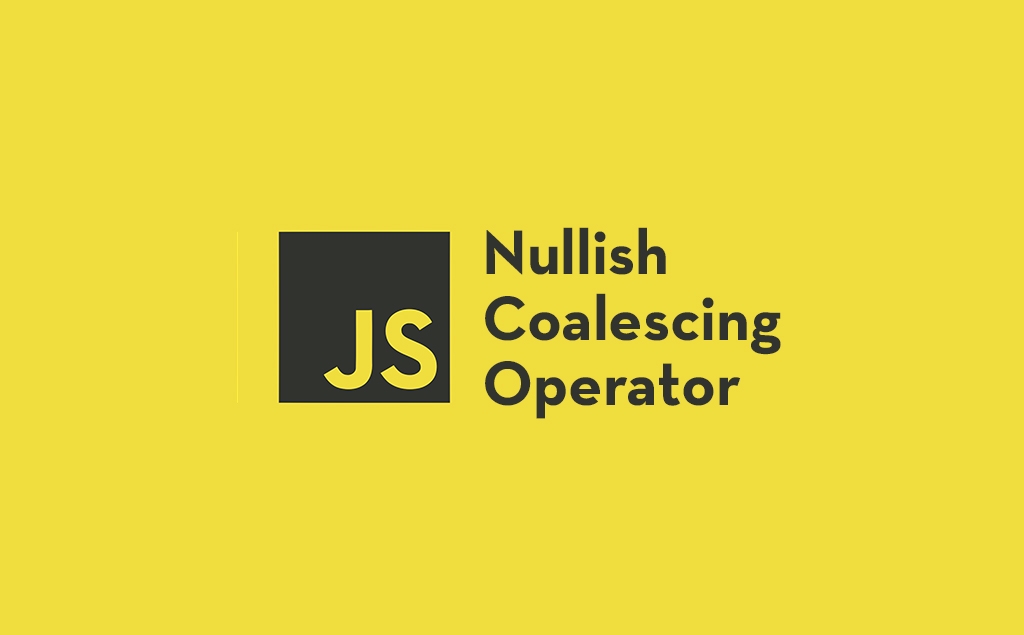
Nullish Coalescing Operator '??' in JavaScript and TypeScript
The nullish coalescing operator (??) is a useful tool in JavaScript and TypeScript for handling null and undefined values. This operator helps provide default values when a variable is null or undefined, making your code more robust and easier to read.
What is the Nullish Coalescing Operator?
The nullish coalescing operator is written as ??. It returns the first argument if it is not null or undefined. Otherwise, it returns the second argument.
Syntax:
let result = a ?? b;
This is equivalent to:
let result = (a !== null && a !== undefined) ? a : b;
How Does It Work?
The ?? operator checks if the value on the left side is null or undefined. If it is, it returns the value on the right side. If the value on the left side is defined (not null or undefined), it returns that value.
Practical Examples
Example 1: Fallback for User Input
Imagine you want to ensure user input has a fallback value in case the user doesn't provide one:
JavaScript:
function getUserName(input) {
return input ?? "Guest";
}
console.log(getUserName(null)); // Output: "Guest"
console.log(getUserName(undefined)); // Output: "Guest"
console.log(getUserName("Alice")); // Output: "Alice"
TypeScript:
function getUserName(input: string | null | undefined): string {
return input ?? "Guest";
}
console.log(getUserName(null)); // Output: "Guest"
console.log(getUserName(undefined)); // Output: "Guest"
console.log(getUserName("Alice")); // Output: "Alice"
Example 2: Configuration Settings
Suppose you have configuration settings where some values might not be set. You want to ensure that default values are used:
JavaScript:
function getConfig(config) {
return {
host: config.host ?? "localhost",
port: config.port ?? 8080,
timeout: config.timeout ?? 3000
};
}
let userConfig = { host: "example.com" };
console.log(getConfig(userConfig));
// Output: { host: "example.com", port: 8080, timeout: 3000 }
TypeScript:
interface Config {
host?: string;
port?: number;
timeout?: number;
}
function getConfig(config: Config): Config {
return {
host: config.host ?? "localhost",
port: config.port ?? 8080,
timeout: config.timeout ?? 3000
};
}
let userConfig: Config = { host: "example.com" };
console.log(getConfig(userConfig));
// Output: { host: "example.com", port: 8080, timeout: 3000 }
Example 3: Nested Nullish Coalescing
Handling multiple layers of potential null or undefined values in an object structure:
JavaScript:
let user = {
name: null,
address: {
street: undefined,
city: "New York"
}
};
let street = user.address?.street ?? "Unknown Street";
let city = user.address?.city ?? "Unknown City";
console.log(street); // Output: "Unknown Street"
console.log(city); // Output: "New York"
TypeScript:
interface Address {
street?: string;
city?: string;
}
interface User {
name?: string | null;
address?: Address;
}
let user: User = {
name: null,
address: {
street: undefined,
city: "New York"
}
};
let street: string = user.address?.street ?? "Unknown Street";
let city: string = user.address?.city ?? "Unknown City";
console.log(street); // Output: "Unknown Street"
console.log(city); // Output: "New York"
Comparison with ||
The || operator returns the first truthy value, whereas ?? returns the first defined value (null or undefined).
Example: Handling Zero Values
JavaScript:
let height = 0;
console.log(height || 100); // Output: 100 (0 is falsy)
console.log(height ?? 100); // Output: 0 (0 is defined)
TypeScript:
let height: number = 0;
console.log(height || 100); // Output: 100 (0 is falsy)
console.log(height ?? 100); // Output: 0 (0 is defined)
The || operator treats 0 as falsy and returns 100, while ?? treats 0 as a valid value and returns 0.
Operator Precedence
The precedence of ?? is similar to ||. This means it is evaluated before assignment (=) and the conditional operator (?:), but after most arithmetic operations like + and *.
Example: Correct Precedence with Parentheses
JavaScript:
let height = null;
let width = null;
// Correct
let area = (height ?? 100) * (width ?? 50); // 5000
console.log(area);
// Incorrect without parentheses
area = height ?? 100 * width ?? 50;
console.log(area); // NaN because 100 * width is evaluated first, resulting in null ?? NaN
TypeScript:
let height: number | null = null;
let width: number | null = null;
// Correct
let area: number = (height ?? 100) * (width ?? 50); // 5000
console.log(area);
// Incorrect without parentheses
area = height ?? 100 * width ?? 50;
console.log(area); // NaN because 100 * width is evaluated first, resulting in null ?? NaN
Safety with ?? and && or ||
JavaScript and TypeScript do not allow mixing ?? with && or || without using parentheses to specify precedence explicitly. This is to prevent unexpected behavior.
Example: Using Parentheses for Safety
JavaScript:
let x = (1 && 2) ?? 3; // Correct usage with parentheses
console.log(x); // Output: 2
// let y = 1 && 2 ?? 3; // Syntax error without parentheses
TypeScript:
let x: number = (1 && 2) ?? 3; // Correct usage with parentheses
console.log(x); // Output: 2
// let y: number = 1 && 2 ?? 3; // Syntax error without parentheses
Summary
The nullish coalescing operator ??
is a concise and powerful tool for handling null
or undefined
values:
Default Value Assignment:
height = height ?? 100;
assigns100
ifheight
isnull
orundefined
.
Precedence:
- Similar to
||
, but consider using parentheses to avoid issues in complex expressions.
Compatibility:
- Do not mix
??
with&&
or||
without using parentheses.
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com