Posted At: Aug 03, 2024 - 192 Views
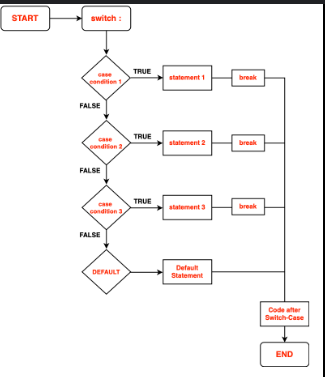
The "switch" Statement
A switch statement can replace multiple if checks, providing a more descriptive way to compare a value with multiple variants.
Syntax
The switch statement has one or more case blocks and an optional default block.
switch(x) {
case 'value1': // if (x === 'value1')
...
[break]
case 'value2': // if (x === 'value2')
...
[break]
default:
...
[break]
}
- The value of
x
is checked for strict equality to each case value. - If a match is found, the code in the corresponding case block is executed until the nearest break or the end of the switch statement.
- If no case matches, the default block (if present) is executed.
JavaScript Example:
let fruit = "orange";
switch (fruit) {
case "banana":
console.log("I am a banana.");
break;
case "apple":
console.log("I am an apple.");
break;
case "orange":
console.log("I am an orange.");
break;
default:
console.log("I am an unknown fruit.");
}
Explanation: Here, fruit
is "orange". The switch statement compares fruit
with each case:
- It skips "banana" and "apple".
- It matches "orange" and executes the corresponding code.
- The break statement prevents further execution.
TypeScript Example:
let fruit: string = "orange";
switch (fruit) {
case "banana":
console.log("I am a banana.");
break;
case "apple":
console.log("I am an apple.");
break;
case "orange":
console.log("I am an orange.");
break;
default:
console.log("I am an unknown fruit.");
}
Explanation: Similar to the JavaScript example, fruit
is "orange". The switch statement compares fruit
with each case, matches "orange", and executes the corresponding code.
Example Without Break
If the break statement is omitted, execution continues to the next case.
JavaScript Example:
let fruit = "orange";
switch (fruit) {
case "banana":
console.log("I am a banana.");
case "apple":
console.log("I am an apple.");
case "orange":
console.log("I am an orange.");
default:
console.log("I am an unknown fruit.");
}
Explanation: Without break statements, the switch statement continues to execute the next cases even if a match is found.
TypeScript Example:
let fruit: string = "orange";
switch (fruit) {
case "banana":
console.log("I am a banana.");
case "apple":
console.log("I am an apple.");
case "orange":
console.log("I am an orange.");
default:
console.log("I am an unknown fruit.");
}
Explanation: Without break statements, the switch statement continues to execute the next cases even if a match is found.
Any Expression as Switch/Case Argument
Both switch and case can have arbitrary expressions.
JavaScript Example:
let number = "2";
let addition = 1;
switch (+number) {
case addition + 1:
console.log("This runs because +number is 2, which equals addition + 1.");
break;
default:
console.log("This doesn't run.");
}
Explanation: Here, +number
converts the string "2" to the number 2, which is compared with addition + 1
(which is also 2).
TypeScript Example:
let number: string = "2";
let addition: number = 1;
switch (+number) {
case addition + 1:
console.log("This runs because +number is 2, which equals addition + 1.");
break;
default:
console.log("This doesn't run.");
}
Explanation: Here, +number
converts the string "2" to the number 2, which is compared with addition + 1
(which is also 2).
Grouping of Cases
Cases can be grouped to share the same code.
JavaScript Example:
let day = "Tuesday";
switch (day) {
case "Monday":
console.log("Start of the work week.");
break;
case "Tuesday":
case "Wednesday":
case "Thursday":
console.log("Midweek days.");
break;
case "Friday":
console.log("End of the work week.");
break;
default:
console.log("Weekend.");
}
Explanation: Cases "Tuesday", "Wednesday", and "Thursday" are grouped together, sharing the same code.
TypeScript Example:
let day: string = "Tuesday";
switch (day) {
case "Monday":
console.log("Start of the work week.");
break;
case "Tuesday":
case "Wednesday":
case "Thursday":
console.log("Midweek days.");
break;
case "Friday":
console.log("End of the work week.");
break;
default:
console.log("Weekend.");
}
Explanation: Cases "Tuesday", "Wednesday", and "Thursday" are grouped together, sharing the same code.
Strict Equality Check
The equality check in a switch statement is always strict (===).
JavaScript Example:
let arg = prompt("Enter a number:");
switch (arg) {
case '1':
case '2':
console.log('One or two');
break;
case '3':
console.log('Three');
break;
case 4:
console.log('Never executes!');
break;
default:
console.log('An unknown value');
}
Explanation: The prompt input is a string. Therefore, case 4 never matches because it’s not strictly equal to the string "4".
TypeScript Example:
let arg: string = prompt("Enter a number:");
switch (arg) {
case '1':
case '2':
console.log('One or two');
break;
case '3':
console.log('Three');
break;
case 4:
console.log('Never executes!');
break;
default:
console.log('An unknown value');
}
Explanation: The prompt input is a string. Therefore, case 4 never matches because it’s not strictly equal to the string "4".
Summary
- The switch statement can simplify multiple if checks.
- The switch evaluates the expression and executes the corresponding case code if a match is found.
- The break statement is used to terminate a case block.
- The default block executes if no case matches.
- Switch and case can accept arbitrary expressions.
- Grouping cases allows them to share code.
- The equality check is strict, meaning the types must match.
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com