Posted At: Jul 14, 2024 - 382 Views
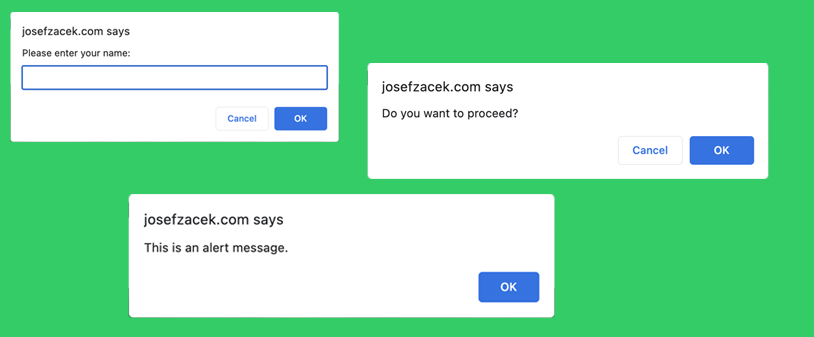
Interaction: alert, prompt, confirm
When working in the browser, JavaScript provides several ways to interact with the user. Three commonly used functions are alert
, prompt
, and confirm
.
1. alert
The alert
function displays a message to the user in a modal window. The user must click "OK" to proceed.
JavaScript Example:
alert("Hello, welcome to our website!");
TypeScript Example:
alert("Hello, welcome to our website!");
- Description: Displays a modal window with a message.
- Modal Window: The user cannot interact with the rest of the page until the alert is dismissed by clicking "OK".
2. prompt
The prompt
function displays a message and requests input from the user. It provides an input field where the user can type a response.
Syntax:
let result = prompt(title, [default]);
let result: string | null = prompt(title, default);
- title: The text to display in the prompt window.
- default: (Optional) The initial value in the input field.
JavaScript Example:
let name = prompt("What is your name?", "Guest");
if (name !== null) {
alert(`Hello, ${name}!`);
} else {
alert("You didn't enter your name.");
}
TypeScript Example:
let name: string | null = prompt("What is your name?", "Guest");
if (name !== null) {
alert(`Hello, ${name}!`);
} else {
alert("You didn't enter your name.");
}
- Behavior: The function returns the text entered by the user or
null
if the user cancels the input.
Note for Internet Explorer:
Always provide a default value to avoid seeing "undefined" in the input field.
Example for IE:
let test = prompt("Test", "");
let test: string | null = prompt("Test", "");
3. confirm
The confirm
function displays a message and asks the user to confirm or cancel.
Syntax:
let result = confirm(question);
let result: boolean = confirm(question);
- question: The text to display in the confirm window.
JavaScript Example:
let isBoss = confirm("Are you the boss?");
if (isBoss) {
alert("Welcome, Boss!");
} else {
alert("You are not the boss.");
}
TypeScript Example:
let isBoss: boolean = confirm("Are you the boss?");
if (isBoss) {
alert("Welcome, Boss!");
} else {
alert("You are not the boss.");
}
- Behavior: The function returns
true
if the user clicks "OK" andfalse
if the user clicks "Cancel".
Summary
alert
- Usage: To show a message.
- Returns: Nothing.
prompt
- Usage: To get input from the user.
- Returns: The input text or
null
if canceled.
confirm
- Usage: To ask for confirmation.
- Returns:
true
if "OK" is clicked,false
if "Cancel" is clicked.
Important Notes
- Modal Nature: All these functions create modal windows, meaning the user must interact with them before returning to the page.
- Browser Dependent: The exact appearance and placement of these windows depend on the browser and cannot be customized.
Example Use Case
Let's create a simple interaction flow using these functions in both JavaScript and TypeScript:
JavaScript Example:
alert("Welcome to our website!");
let age = prompt("How old are you?", "18");
if (age !== null) {
let isAdult = confirm("Are you sure you are an adult?");
if (isAdult) {
alert("Access granted");
} else {
alert("Access denied");
}
} else {
alert("Please provide your age");
}
TypeScript Example:
alert("Welcome to our website!");
let age: string | null = prompt("How old are you?", "18");
if (age !== null) {
let isAdult: boolean = confirm("Are you sure you are an adult?");
if (isAdult) {
alert("Access granted");
} else {
alert("Access denied");
}
} else {
alert("Please provide your age");
}
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com