Posted At: Jul 08, 2024 - 578 Views
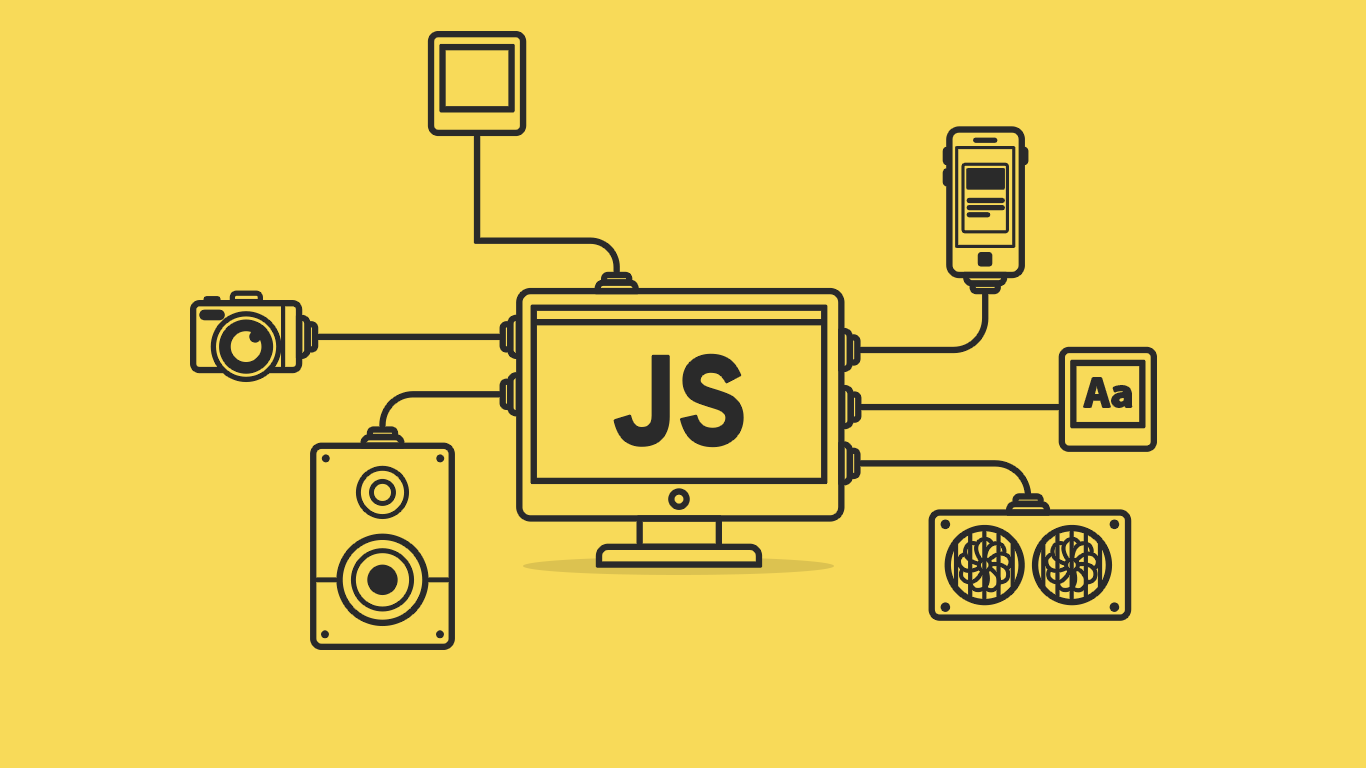
Master JavaScript: From Basics to Advanced
The main course is structured into four parts: JavaScript Tutorial, Browser DOM, JavaScript with DSA, and Logic & Real World Project Building. Additionally, there are specialized thematic articles.
Table of Contents
- JavaScript Tutorial
- Browser Dom
- JavaScript With DSA
- Logic & Real World Project Building
Part 1: The JavaScript Language
- In this course, we start from the basics of JavaScript and progress to advanced topics like OOP
- Our focus is on the core language, keeping environment-specific details to a minimum.
- Building a Strong Foundation: Mastering JavaScript Fundamentals
An introduction & Requirements
JavaScript Fundamentals
- Introduction: Hello, World!
- Structuring Your Code
- Using "use strict" Mode
- Understanding Variables
- Exploring Data Types
- User Interactions: Alert, Prompt, Confirm
- Type Conversions
- Basic Operators and Math
- Making Comparisons
- Conditional Branching: if, '?'
- Logical Operators
- Nullish Coalescing Operator '??'
- Utilizing Loops: while and for
- The "switch" Statement
- Functions: An Overview
- Function Expressions
- Arrow Functions: The Basics
- Special Features in JavaScript
Exploring Data Types
- Primitive Methods
- Numbers
- Strings
- Arrays
- Array Methods
- Iterables
- Map and Set
- WeakMap and WeakSet
- Object Keys, Values, Entries
- Destructuring Assignment
- Date and Time
- JSON Methods and toJSON
Advanced working with functions
- Recursion and the Call Stack
- Rest Parameters and Spread Syntax
- Variable Scope and Closures
- The Legacy of "var"
- The Global Object
- Function Objects and Named Function Expressions (NFE)
- The "new Function" Syntax
- Scheduling with setTimeout and setInterval
- Decorators and Function Forwarding with call/apply
- Function Binding
- Revisited: Arrow Functions
Objects & Object Properties Configuration
- Introduction to Objects
- Object References and Copying
- Garbage Collection
- Object Methods and the "this" Keyword
- Constructors and the "new" Operator
- Optional Chaining (?.)
- The Symbol Type
- Object to Primitive Conversion
- Object Property flags and descriptors
- Getters and setters
Understanding Prototypal Inheritance
- Understanding Prototypal Inheritance
- The
F.prototype
Property - Working with Native Prototypes
- Prototype Methods and Objects without
__proto__
Class Of JavaScript ( OOPs)
- Basic Class Syntax
- Class Inheritance
- Static Properties and Methods
- Private and Protected Properties and Methods
- Extending Built-in Classes
- Class Checking: "instanceof"
Mixins
Promises, Async/Await
- Introduction to Callbacks
- Promises
- Promise Chaining
- Error Handling with Promises
- Promise API
- Promisification
- Microtasks
- Async/Await
Generators, Advanced Iteration
- Generators
- Async Iteration and Generators
Modules
- Introduction to Modules
- Export and Import
- Dynamic Imports
Miscellaneous
- Proxy and Reflect
- Eval: Running Code Strings
- Currying
- Reference Type
- BigInt
- Unicode and String Internals
- WeakRef and FinalizationRegistry
Error Handling
- Basic Error Handling with "try...catch"
- Custom Errors and Extending Error
Code quality
- Writing Effective Comments
- Browser Debugging Techniques
- Best Practices for Coding Style
- Ninja Coding Techniques
- Automated Testing with Mocha
- Polyfills and Transpilers
Next → Browser Dom With JavaScript
Call to Action
How do you think AI will shape the future of technology? Share your thoughts in the comments below. For more insights into the latest tech trends, visit our website PlambIndia and stay updated with our blog.
Follow Us
Stay updated with our latest projects and insights by following us on social media:
- LinkedIn: PlambIndia Software Solutions
- PlambIndia: Plambindia Software Solution.
- WhatsApp Number: +91 87663 78125
- Email: contact@plambIndia.com , kuldeeptrivedi456@gmail.com
Become a Client
Explore our diverse range of services and find the perfect solution tailored to your needs. Select a category below to learn more about how we can help transform your business.
Kuldeep Trivedi
plot no 1 / 2 suraj mall compound mal compound
+918766378125
contact@plambindia.com